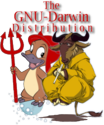
|
Main Page
Widgets
Namespaces
Book
Glib::Date Class ReferenceJulian calendar date.
More...
List of all members.
|
Public Types |
typedef guint8 | Day |
typedef guint16 | Year |
enum | Month {
BAD_MONTH,
JANUARY,
FEBRUARY,
MARCH,
APRIL,
MAY,
JUNE,
JULY,
AUGUST,
SEPTEMBER,
OCTOBER,
NOVEMBER,
DECEMBER
} |
enum | Weekday {
BAD_WEEKDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
} |
enum | DMY {
DAY,
MONTH,
YEAR
} |
Public Methods |
| Date () |
| Date (Day day, Month month, Year year) |
| Date (guint32 julian_day) |
void | clear () |
void | set_parse (const Glib::ustring& str) |
void | set_time (GTime time) |
void | set_month (Month month) |
void | set_day (Day day) |
void | set_year (Year year) |
void | set_dmy (Day day, Month month, Year year) |
void | set_julian (guint32 julian_day) |
Date& | add_days (int n_days) |
Date& | subtract_days (int n_days) |
Date& | add_months (int n_months) |
Date& | subtract_months (int n_months) |
Date& | add_years (int n_years) |
Date& | subtract_years (int n_years) |
int | days_between (const Date& rhs) const |
int | compare (const Date& rhs) const |
Date& | clamp (const Date& min_date, const Date& max_date) |
void | order (Date& other) |
Weekday | get_weekday () const |
Month | get_month () const |
Year | get_year () const |
Day | get_day () const |
guint32 | get_julian () const |
unsigned int | get_day_of_year () const |
unsigned int | get_monday_week_of_year () const |
unsigned int | get_sunday_week_of_year () const |
bool | is_first_of_month () const |
bool | is_last_of_month () const |
Glib::ustring | format_string (const Glib::ustring& format) const |
| Convert date to string.
|
void | to_struct_tm (struct tm& dest) const |
bool | valid () const |
Static Public Methods |
guint8 | get_days_in_month (Month month, Year year) |
guint8 | get_monday_weeks_in_year (Year year) |
guint8 | get_sunday_weeks_in_year (Year year) |
bool | is_leap_year (Year year) |
bool | valid_day (Day day) |
bool | valid_month (Month month) |
bool | valid_year (Year year) |
bool | valid_weekday (Weekday weekday) |
bool | valid_julian (guint32 julian_day) |
bool | valid_dmy (Day day, Month month, Year year) |
Static Public Attributes |
const Day | BAD_DAY = 0 |
const Year | BAD_YEAR = 0 |
const guint32 | BAD_JULIAN = 0 |
Related Functions |
(Note that these are not member functions.)
|
bool | operator== (const Date& lhs, const Date& rhs) |
bool | operator!= (const Date& lhs, const Date& rhs) |
bool | operator< (const Date& lhs, const Date& rhs) |
bool | operator> (const Date& lhs, const Date& rhs) |
bool | operator<= (const Date& lhs, const Date& rhs) |
bool | operator>= (const Date& lhs, const Date& rhs) |
Detailed Description
Julian calendar date.
Member Typedef Documentation
typedef guint8 Glib::Date::Day
|
|
typedef guint16 Glib::Date::Year
|
|
Constructor & Destructor Documentation
Glib::Date::Date |
( |
guint32 |
julian_day |
) |
[explicit] |
|
Member Function Documentation
Date& Glib::Date::add_days |
( |
int |
n_days |
) |
|
|
Date& Glib::Date::add_months |
( |
int |
n_months |
) |
|
|
Date& Glib::Date::add_years |
( |
int |
n_years |
) |
|
|
Date& Glib::Date::clamp |
( |
const Date& |
min_date, |
|
|
const Date& |
max_date |
|
) |
|
|
void Glib::Date::clear |
( |
|
) |
|
|
int Glib::Date::compare |
( |
const Date& |
rhs |
) |
const |
|
int Glib::Date::days_between |
( |
const Date& |
rhs |
) |
const |
|
|
Convert date to string.
- Parameters:
-
format | A format string as used by strftime(), in UTF-8 encoding. Only date formats are allowed, the result of time formats is undefined. |
- Returns:
- The formatted date string.
- Exceptions:
-
|
Day Glib::Date::get_day |
( |
|
) |
const |
|
unsigned int Glib::Date::get_day_of_year |
( |
|
) |
const |
|
guint8 Glib::Date::get_days_in_month |
( |
Month |
month, |
|
|
Year |
year |
|
) |
[static] |
|
guint32 Glib::Date::get_julian |
( |
|
) |
const |
|
unsigned int Glib::Date::get_monday_week_of_year |
( |
|
) |
const |
|
guint8 Glib::Date::get_monday_weeks_in_year |
( |
Year |
year |
) |
[static] |
|
Month Glib::Date::get_month |
( |
|
) |
const |
|
unsigned int Glib::Date::get_sunday_week_of_year |
( |
|
) |
const |
|
guint8 Glib::Date::get_sunday_weeks_in_year |
( |
Year |
year |
) |
[static] |
|
Weekday Glib::Date::get_weekday |
( |
|
) |
const |
|
Year Glib::Date::get_year |
( |
|
) |
const |
|
bool Glib::Date::is_first_of_month |
( |
|
) |
const |
|
bool Glib::Date::is_last_of_month |
( |
|
) |
const |
|
bool Glib::Date::is_leap_year |
( |
Year |
year |
) |
[static] |
|
void Glib::Date::order |
( |
Date& |
other |
) |
|
|
void Glib::Date::set_day |
( |
Day |
day |
) |
|
|
void Glib::Date::set_dmy |
( |
Day |
day, |
|
|
Month |
month, |
|
|
Year |
year |
|
) |
|
|
void Glib::Date::set_julian |
( |
guint32 |
julian_day |
) |
|
|
void Glib::Date::set_month |
( |
Month |
month |
) |
|
|
void Glib::Date::set_time |
( |
GTime |
time |
) |
|
|
void Glib::Date::set_year |
( |
Year |
year |
) |
|
|
Date& Glib::Date::subtract_days |
( |
int |
n_days |
) |
|
|
Date& Glib::Date::subtract_months |
( |
int |
n_months |
) |
|
|
Date& Glib::Date::subtract_years |
( |
int |
n_years |
) |
|
|
void Glib::Date::to_struct_tm |
( |
struct tm& |
dest |
) |
const |
|
bool Glib::Date::valid |
( |
|
) |
const |
|
bool Glib::Date::valid_day |
( |
Day |
day |
) |
[static] |
|
bool Glib::Date::valid_dmy |
( |
Day |
day, |
|
|
Month |
month, |
|
|
Year |
year |
|
) |
[static] |
|
bool Glib::Date::valid_julian |
( |
guint32 |
julian_day |
) |
[static] |
|
bool Glib::Date::valid_month |
( |
Month |
month |
) |
[static] |
|
bool Glib::Date::valid_weekday |
( |
Weekday |
weekday |
) |
[static] |
|
bool Glib::Date::valid_year |
( |
Year |
year |
) |
[static] |
|
Friends And Related Function Documentation
bool operator!= |
( |
const Date& |
lhs, |
|
|
const Date& |
rhs |
|
) |
[related] |
|
bool operator< |
( |
const Date& |
lhs, |
|
|
const Date& |
rhs |
|
) |
[related] |
|
bool operator<= |
( |
const Date& |
lhs, |
|
|
const Date& |
rhs |
|
) |
[related] |
|
bool operator== |
( |
const Date& |
lhs, |
|
|
const Date& |
rhs |
|
) |
[related] |
|
bool operator> |
( |
const Date& |
lhs, |
|
|
const Date& |
rhs |
|
) |
[related] |
|
bool operator>= |
( |
const Date& |
lhs, |
|
|
const Date& |
rhs |
|
) |
[related] |
|
Member Data Documentation
const Day Glib::Date::BAD_DAY = 0 [static]
|
|
const guint32 Glib::Date::BAD_JULIAN = 0 [static]
|
|
const Year Glib::Date::BAD_YEAR = 0 [static]
|
|
The documentation for this class was generated from the following file:
Generated for gtkmm by
Doxygen 1.3-rc1 © 1997-2001
|